UART is an acronym for Universal Asynchronous Receiver/Transmitter. UART is one of the most used device to device communication protocols. In UART the data format and communication speed are configurable. UART supports asynchronous serial communication.
Serial UART Communication
Serial UART is the serial communication protocol used by Arduino to communicate with other devices. It is a method of sending data in which the data is sent bit by bit.
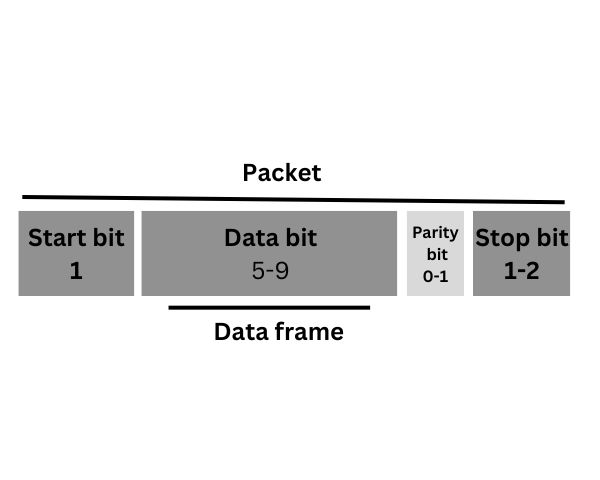
UART Parameters
The UART has some settings that are needed to be the same on both devices to establish proper communication between them. These UART settings are the baud rate, data length, parity bit, number of stop bits, and flow control.
Bit Rate & Baud Rate
The rate of data transfer in serial data communication protocol is calculated in bps (bits per second). A widely used terminology for bps is the baud rate, which means a number of changes in signal per second. Here in the serial UART communication signals are in bits, therefore bit rate = baud rate.
Data Length:-
Data length refers to the number of bits per byte of data.
Parity Bit
The parity bit is the bit that is added to the transmitted data that tells the receiver if the number of 1s in the data transmitted is odd or even.
- ODD – the parity bit is ‘1’ if there is an odd number of 1’s in the data frame
- EVEN – the parity bit is ‘0’ if there is an even number of 1’s in the data frame.
Number Of Stop Bits:-
The UART devices can use none, one or two-stop bits which are used to mark the end of a set of bits often called packets transmitted.
Flow Control:-
Flow Control is the method that is used to avoid the risk of losing data while transmitting the data over UART.
The UART device uses special characters as flow control to start/stop transmission if it recognizes any data loss.
A serial bus with two terminals is used for UART communication which is TX (D1) and RX(Do).
- TX is used for transmitting the data
- RX is used for receiving the data.
We have to keep in mind that UART are specific for devices. It means that if we want to establish serial communication using UART between two Arduino boards(A and B).
After successfully connecting the two Arduino boards we will start UART serial communication between them. We will use a master-slave configuration for this purpose. One of the Arduino boards will transfer or transmit data through TX pin which will act as master and the second Arduino board which will receive instructions will act as Slave.
Functions Used for Arduino Serial Communication:-
UART pins of arduino uno
Serial.begin(Baud rate):-
This function is used for initiating serial communication with the baud rate.
For example:-
Serial.begin(9600)
Serial.print (data, format type (optional)):-
This function sends the ASCII character on the serial port so humans are unable to read it easily. This function converts the data to ASCII characters and then sends it for print.
For example:-
Serial.print (123);
Serial.print (“123”);
Serial.print (12, HEX);
Serial.println (data, format type (optional)):-
This function is used to send the ASCII character on the serial port followed by a carriage return.
Serial.write():-
This function is used to write binary data to the serial port so that it returns the number of bytes written.
For example:-
Serial.write (65);
Serial.available ():-
This function is used to return the number of bytes available to read.
Serial.read():-
This function is used to read the received data serially.
For example:-
received_data = Serial.read()
Serial.readString():-
This function is used to read the received string.
For example:-
String received_data = Serial.readString()
Working Of Universal Asynchronous Receiver/Transmitter Arduino:-
When the computer sends data to the Arduino, this process is done through USB.
Once the data is transferred this USB signal is then converted into serial form and transmitted to the UART. The received data is then placed in a buffer, which is a memory storage used to hold the data temporarily.
Only three wires are required for serial communication and they are:
- TX: The line for transmitting data
- RX: The line for receiving data
- GND: Common ground
The transmission line is used to send data to the slave device. Therefore, the sender device’s TX line needs to be connected to the slave device’s RX line.
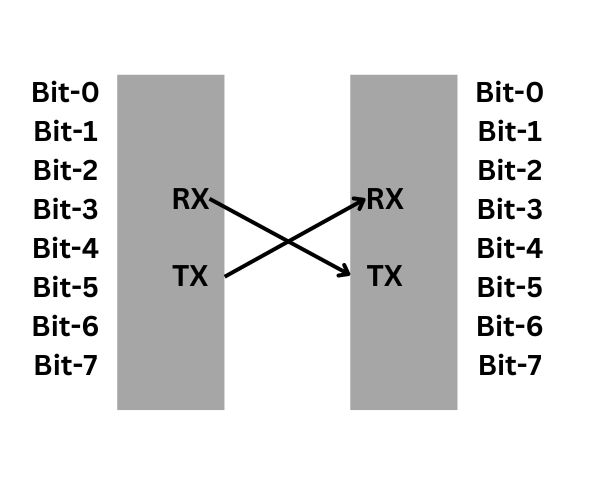
In UART serial communication, both devices can send and receive data at the same time, which means it is capable of full-duplex communication.
As its name suggests, UART transmits data asynchronously, which means there is no external clock line driving the bits.
The start bit indicates the start of a data word and is used to synchronize the transmitter and receiver. This is always 0.
The stop bit indicates when the last data bit has been sent. Modern devices use a 1 stop bit.
The data packet, usually 5 to 9 bits in length, is the information to be transferred. This information is in the form of binary data bits.
The parity bit is used for error detection via a simple error-checking algorithm that is now rarely used.
Program For Transmitting Data Serially To Computer Using Arduino:-
The code for transmitting data serially to the computer using Arduino is as follows:-
void setup() {
Serial.begin (9600); //initiate serial communication with 9600 baud rate
}
void loop() {
Serial.println("hello"); //send data to computer
delay(1000);
}
Program For Reading data:-
The code for reading data serially from the pc and displaying it on a serial monitor is as follows:-
char received_data; //variable to store read data
void setup() {
Serial.begin(9600);
}
void loop() {
if(Serial.available()>0) //check for any data received
{
received_data = Serial.read(); //read received data
Serial.print("received data is: ");
Serial.println(received_data); //display received data
}
}
Program for Reading transmitted String from PC using Arduino:-
In this, the master Arduino will continuously send or transmit a string of data to the slave Arduino.
Code for Reading transmitted String from PC using Arduino is as follows:-
String receive_buffer;
void setup() {
Serial.begin(9600);
}
void loop() {
if(Serial.available()>0) //check for any data received
{
receive_buffer = Serial.readString(); //read received data
Serial.print("received data is: ");
Serial.println(receive_buffer); //display received data
}
}
Program for on and off of LED:-
char received_data;
void setup() {
Serial.begin(9600);
pinMode(13, OUTPUT);
}
void loop() {
if(Serial.available()>0){
//read serial data
received_data = (char)Serial.read();
Serial.print("received data is: ");
//check for received command for action
if(received_data=='1'){
digitalWrite(13, HIGH);
Serial.println(received_data);
}
else if(received_data=='0'){
digitalWrite(13, LOW);
Serial.println(received_data);
}
else
Serial.println(" invalid");
}
}
Advantages And Disadvantages Of Using UART in Arduino:-
The advantages of using UART in Arduino are as follows:-
- The UART communication protocol is Simple to operate and use with Arduino.
- No clock is needed in UART communication.
- UART with Arduino is a widely used method by Arduino users and yet it is a very desirable choice for many Arduino projects.
The disadvantages of using UART in Arduino are as follows:-
- UART has a Lower speed compared to that I2C and SPI.
- The Baud rates of each UART must be within 10% of each other to prevent data loss.
- UART Cannot use multiple master-slave systems like I2C and SPI.
- The size of the data frame is limited to only 9 bits.
Frequently Asked Questions:-
- How does UART work without a clock?
The UART interface does not need a clock signal to synchronize the transmitter and receiver devices because it transmits data asynchronously.
- How much current does UART use?
UART generally uses 55 micro-ampere(uA) current.
- Can UART be a full duplex?
The UART can be configured for Full Duplex, Half Duplex, RX-only or TX-only versions
- Why is UART called universal?
The universal in name of UART refers to the configurability of both the data format and the speeds at which it is transmitted/received.
- Who Invented UART?
The first UART was invented by Gordon Bell of DEC.
3 thoughts on “1 article all about UART (Universal Asynchronous Receiver/Transmitter) protocols.”