Have you ever thought about how smartwatches measure the pulse rate? Did you ever desire to make your own pulse rate reader? In this article, we are going to learn about the working of pulse rate sensors and interface it with Arduino?
Material required
For this project, we will require the following material.
Name | Quantity |
Arduino UNO | 1 |
Pulse sensor | 1 |
jumper wires | as per requirement |
USB cable | 1 |
How pulse rate sensor Work
The Optical heart rate sensor emits a green light (~550nm) on the finger and measures the reflected light.
The oxygenated hemoglobin in the arterial blood absorbs more green light. As the blood is pumped through the finger, the amount of reflected light is read by the photosensor.
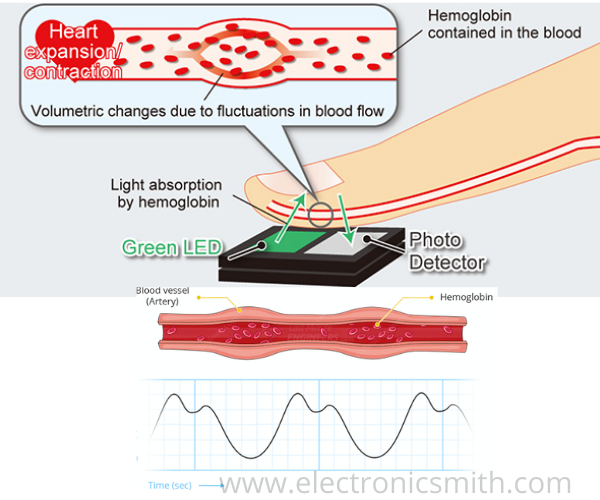
Pulse sensor specification
The front of the sensor has a heart image, this is the place where you have to place your finger there. This side of the sensor also has a green reverse mount LED (565nm) and an APDS light photosensor. (525nm)
The backside of the sensor has MCP6001 Op-Amp and a reverse protected diode.
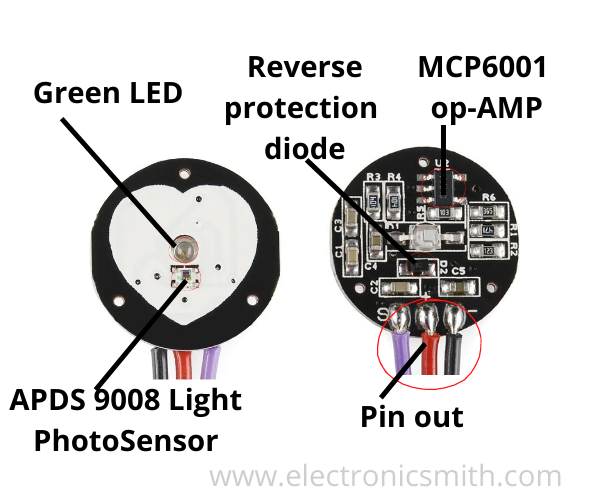
Pin Out
The Heart rate sensor has mainly 3 pins. Vcc, Gnd, and signal.

Pin Name | Function |
Signal(s) | The signal output of the sensor |
Vcc(+) | +5v |
GND(-) | Ground |
Pulse Rate Sensor Connection with Arduino
The Connection of the pulse rate sensor with Arduino is very simple. Two for power and a single signal pin.

The module can power from 3.3v or 5v. The positive pin of the module will be connected with 5v of Arduino. The negative wire of the module will be connected with Arduino GND. The last pin signal() of the module will be connected to Arduino A0.
Arduino | Sensor |
A0 | Signal(s) |
GND | GND |
Vcc | Vcc |
Required library Installation
In order to work with a PulseSensor, you will need to install a library called ‘PulseSensor Playground‘. To install this library go to Sketch > Include Library > Manage Libraries. Search for ‘PulseSensor‘ and install it.
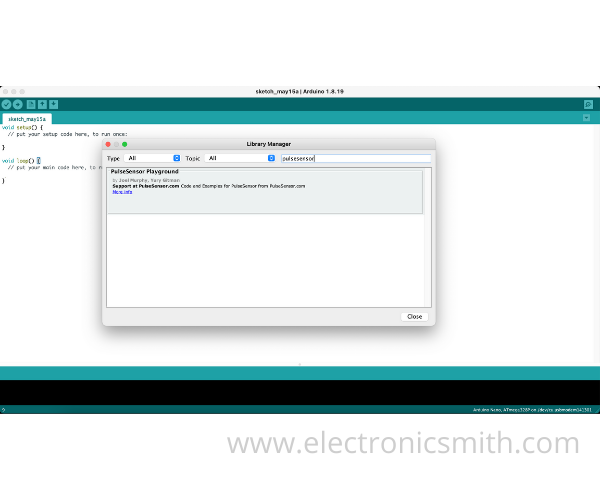
Example Code of PulseSensor
PilseSensor library has a number of example sketches. we’ll go over a few of them here. You can try other examples and develop cool projects.

Here we will work with the most important sketch. To open it Navigate to File > Examples > PulseSensor Playground> GettingStartedProject and open the sketch.
Upload the code to Arduino.
int const PULSE_SENSOR_PIN = 0; // 'S' Signal pin connected to A0
int Signal; // Store incoming ADC data. Value can range from 0-1024
int Threshold = 550; // Determine which Signal to "count as a beat" and which to ignore.
void setup() {
pinMode(LED_BUILTIN,OUTPUT); // Built-in LED will blink to your heartbeat
Serial.begin(9600); // Set comm speed for serial plotter window
}
void loop() {
Signal = analogRead(PULSE_SENSOR_PIN); // Read the sensor value
Serial.println(Signal); // Send the signal value to serial plotter
if(Signal > Threshold){ // If the signal is above threshold, turn on the LED
digitalWrite(LED_BUILTIN,HIGH);
} else {
digitalWrite(LED_BUILTIN,LOW); // Else turn off the LED
}
delay(10);
}
Arduino sketch to measure heart rate (BPM)
In this example, we are going to measure BPM(beats per minute). Again Go to File > Examples > PulseSensor Playground>Getting_BPM_to_Monitor open and upload it to Arduino.
#define USE_ARDUINO_INTERRUPTS true // Set-up low-level interrupts for most acurate BPM math
#include <PulseSensorPlayground.h> // Includes the PulseSensorPlayground Library
const int PulseWire = 0; // 'S' Signal pin connected to A0
const int LED13 = 13; // The on-board Arduino LED
int Threshold = 550; // Determine which Signal to "count as a beat" and which to ignore
PulseSensorPlayground pulseSensor; // Creates an object
void setup() {
Serial.begin(9600);
// Configure the PulseSensor object, by assigning our variables to it
pulseSensor.analogInput(PulseWire);
pulseSensor.blinkOnPulse(LED13); // Blink on-board LED with heartbeat
pulseSensor.setThreshold(Threshold);
// Double-check the "pulseSensor" object was created and began seeing a signal
if (pulseSensor.begin()) {
Serial.println("PulseSensor object created!");
}
}
void loop() {
int myBPM = pulseSensor.getBeatsPerMinute(); // Calculates BPM
if (pulseSensor.sawStartOfBeat()) { // Constantly test to see if a beat happened
Serial.println("♥ A HeartBeat Happened ! "); // If true, print a message
Serial.print("BPM: ");
Serial.println(myBPM); // Print the BPM value
}
delay(20);
}