Hello everyone, I was searching the for a simple RF Transmitter-receiver module guide for use in remote control systems like relay controlling or some basic tasks. Unfortunately, I did not find such a guide. Therefore, I wrote a simple article discussing this transceiver, connecting it to Arduino, and programming it.
Materials Required
Materials | Quantity |
Arduino Board | 2 |
RF Transmitter-Receiver Module | 1 pair |
Jumper Wires | as needed |
Breadboard | 1 |
External Power Supply (9V Battery) | 2 |
Module Specifications
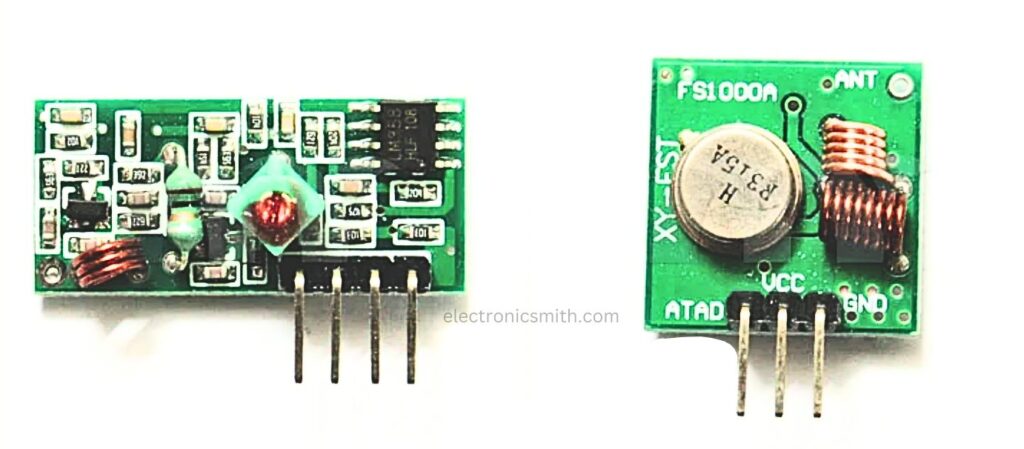
Transmitter:
- Operating voltage: 3V – 12V (for max power use 12V)
- Operating current: max 40mA, min 9mA
- Resonance mode: (SAW)
- Modulation mode: ASK
- Working frequency: 315MHz or 433MHz
- Transmission power: 25mW (315MHz at 12V)
- Frequency error: +150kHz (max)
- Velocity: less than 10Kbps
This module will transmit up to 90m in an open area.
Receiver:
- Working voltage: 5.0VDC +0.5V
- Working current: ≤5.5mA max
- Working method: OOK/ASK
- Working frequency: 315MHz-433.92MHz
- Bandwidth: 2MHz
- Sensitivity: excellent –100dBm (50Ω)
- speed: <9.6Kbps (at 315MHz and -95dBm)
Use of an optional antenna will improve the performance of your wireless communication. A single wire is good enough.
RF 315 433mhz Transmitter Reciever Schematics
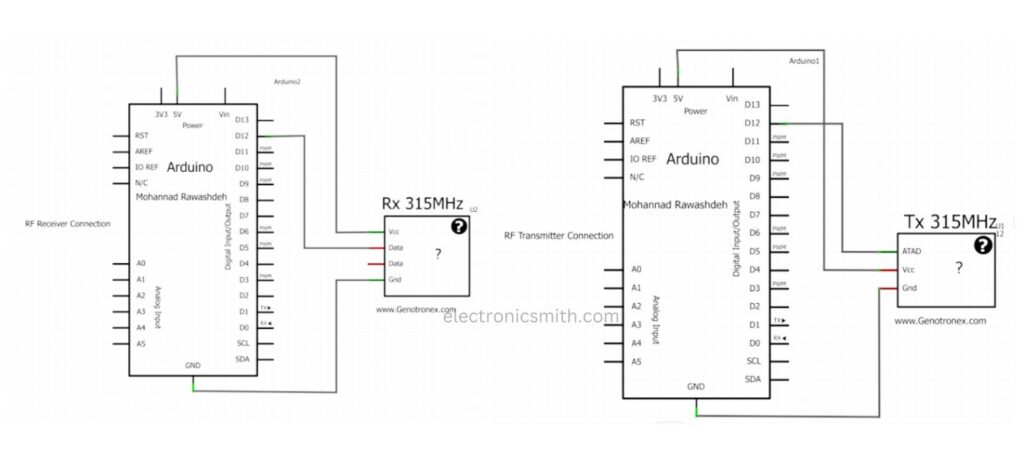
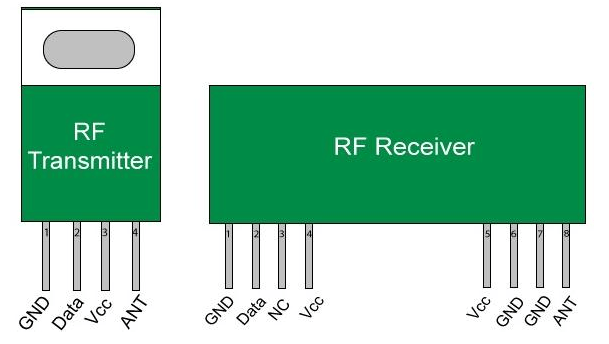
The connection for this module is quite easy.
Transmitter:
- Vcc → 5V
- DATA → D12 (You can change it as you like in the software)
- Gnd → Gnd
Receiver:
- Vcc → 5V
- DATA → D12
- Gnd → Gnd
Arduino Virtual Wire Library
There’s a well-used library for Arduino called VirtualWire developed by Mike McCauley. VirtualWire is an Arduino library offering functionality to send short messages without address, retransmission or acknowledgment, something akin to UDP over wireless using ASK or amplitude shift keying. It supports a range of cheap radio transmitters and receivers. It enables you to simply send and receive “bytes” and strings.
First, download the library from the link, and after extracting the folder, move it to the “Libraries” folder in your Arduino directory. Here’s a simple code that sends character ‘1’ and after 2 seconds sends character ‘0’, and so on.
Code for Transmitter:
#include <VirtualWire.h>
char *controller;
void setup() {
pinMode(13, OUTPUT);
vw_set_ptt_inverted(true); // For DR3100
vw_set_tx_pin(12);
vw_setup(4000); // Speed of data transfer (Kbps)
}
void loop() {
controller = "1";
vw_send((uint8_t *)controller, strlen(controller));
vw_wait_tx(); // Wait until the whole message is sent
digitalWrite(13, HIGH);
delay(2000);
controller = "0";
vw_send((uint8_t *)controller, strlen(controller));
vw_wait_tx(); // Wait until the whole message is sent
digitalWrite(13, LOW);
delay(2000);
}
For receiver:
#include <VirtualWire.h>
void setup() {
vw_set_ptt_inverted(true); // For DR3100
vw_set_rx_pin(12);
vw_setup(4000); // Speed (Bits per second)
pinMode(13, OUTPUT);
vw_rx_start(); // Start the receiver PLL running
}
void loop() {
uint8_t buf[VW_MAX_MESSAGE_LEN];
uint8_t buflen = VW_MAX_MESSAGE_LEN;
if (vw_get_message(buf, &buflen)) { // Non-blocking
if (buf[0] == '1') {
digitalWrite(13, HIGH);
}
if (buf[0] == '0') {
digitalWrite(13, LOW);
}
}
}
One Transmitter, Multi-Receivers
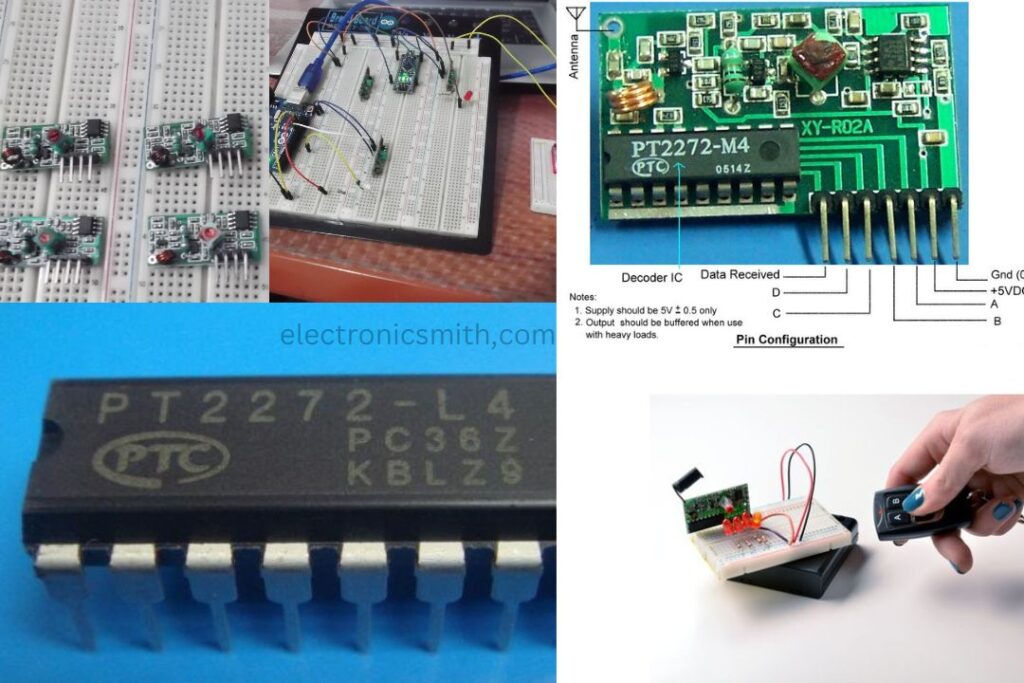
You can connect many receivers to send the data from one master transmitter. In case of more complexity, you might need an Encoder-Decoder circuit.
An Encoder is a circuit that changes a set of signals into a code. A decoder is a circuit that can turn the code back into a set of signals. If you need an Encoder/Decoder IC, you may use PT2262 and PT2272.
Here is an example with 1 master transmitter and 2 receivers. The transmitter sends a command via serial to control an LED.
Transmitter code
#include <VirtualWire.h>
char *controller;
void setup() {
pinMode(13, OUTPUT);
vw_set_ptt_inverted(true); // For DR3100
vw_set_tx_pin(12);
vw_setup(4000); // Speed of data transfer (Kbps)
}
void loop() {
controller = "A1";
vw_send((uint8_t *)controller, strlen(controller));
vw_wait_tx(); // Wait until the whole message is sent
digitalWrite(13, HIGH);
delay(1000);
digitalWrite(13, LOW);
delay(1000);
controller = "B1";
vw_send((uint8_t *)controller, strlen(controller));
vw_wait_tx(); // Wait until the whole message is sent
digitalWrite(13, HIGH);
delay(1000);
digitalWrite(13, LOW);
delay(1000);
}
1st receiver:
#include <VirtualWire.h>
void setup() {
vw_set_ptt_inverted(true); // For DR3100
vw_set_rx_pin(12);
vw_setup(4000); // Bits per second
pinMode(13, OUTPUT);
vw_rx_start(); // Start the receiver PLL running
}
void loop() {
uint8_t buf[VW_MAX_MESSAGE_LEN];
uint8_t buflen = VW_MAX_MESSAGE_LEN;
if (vw_get_message(buf, &buflen)) { // Non-blocking
if ((buf[0] == 'A') && (buf[1] == '1')) {
digitalWrite(13, HIGH);
delay(1000);
}
} else {
digitalWrite(13, LOW);
}
}
2nd receiver
#include <VirtualWire.h>
void setup() {
vw_set_ptt_inverted(true); // For DR3100
vw_set_rx_pin(12);
vw_setup(4000); // Bits per second
pinMode(13, OUTPUT);
vw_rx_start(); // Start the receiver PLL running
}
void loop() {
uint8_t buf[VW_MAX_MESSAGE_LEN];
uint8_t buflen = VW_MAX_MESSAGE_LEN;
if (vw_get_message(buf, &buflen)) { // Non-blocking
if ((buf[0] == 'B') && (buf[1] == '1')) {
digitalWrite(13, HIGH);
delay(1000);
}
} else {
digitalWrite(13, LOW);
}
}
Virtual Wire Inside
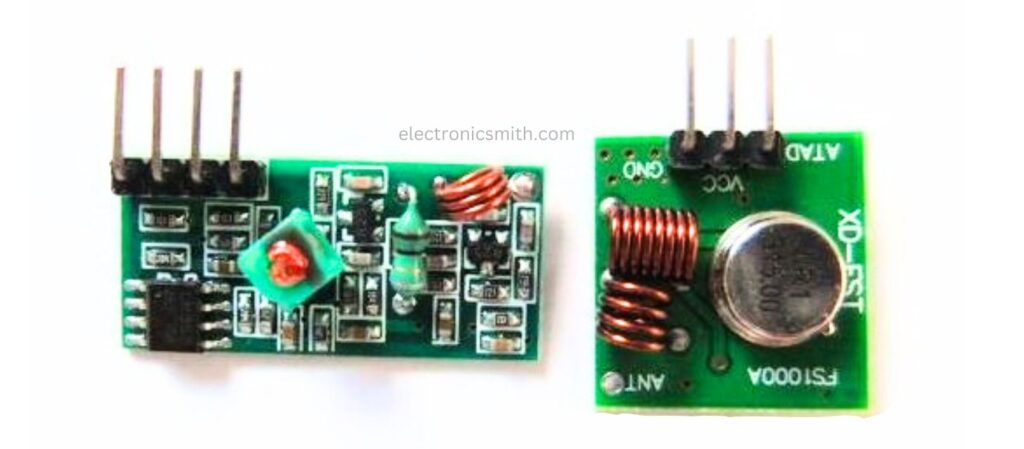
VirtualWire is an Arduino library that sends short messages using ASK (amplitude shift keying) over wireless, like UDP, without retransmitting or acknowledging.
Messages have a preamble, length, and checksum. It uses 4-to-6-bit encoding for DC balance and CRC checksum for message integrity.
Can you use Serial communication? No, because ASK requires burst pulses and good DC balance that UARTs don’t provide well. However, the VirtualWire library works better for ASK wireless communication.
To use the library, include it as follows:
cpp
Copy
Edit
#include <VirtualWire.h>
For transmitting and receiving, use these functions:
vw_set_tx_pin: To select the transmitter pin.
vw_set_rx_pin: To select the receiver pin.
vw_setup: To set the transmission speed.
vw_rx_start: To start the receiver.
vw_wait_tx: To wait until the transmission is done.
vw_have_message: To receive a message.