A flex sensor or bend sensor is a sensor that measures bend or angular deflection. In this article, we will learn about the working of flex sensors, interface them with Arduino, and control a servo motor.
Working of the flex sensor
A flex sensor is basically a resistor that changes its resistance with a bend. The flex sensor consists of a phenolic resin substrate with conductive ink deposited(made of carbon). A segmented conductor is placed on top to form a flexible potentiometer in which resistance changes upon deflection.

Flex sensor
Flex sensors have 2 pins (you can connect them in any direction). Also, sensors are designed to bend in one direction away from ink.
The sensor’s resistance is minimum when it is relaxed or straight and maximum when it is bent.

Connection With Arduino
The connection of the bend sensor with Arduino is quite easy. The only point to remember is to connect a 47kΩ pull-down resistor in series to create a voltage divider circuit.

Code
This code will give you some row numbers depending on the bend of the flex sensor on the serial monitor.
//www.elecrtonicsmith.com check ower website for more projects.
const int flexPin = A0;
void setup()
{
Serial.begin(9600);
}
void loop()
{
int flexValue;
flexValue = analogRead(flexPin);
Serial.print("sensor: ");
Serial.println(flexValue);
delay(20);
}
connection of servo and flex sensor with Arduino
The connection of the servo motor with Arduino is a very simple connect the signal wire of the servo to Arduino pin no. 7. The red wire of the servo to 5v, and the black wire of the servo to GND of Arduino.
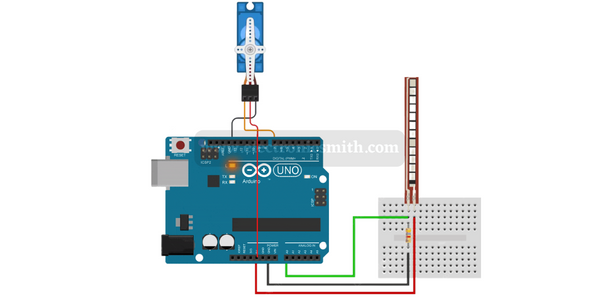
code
Copy the following code and upload it to Arduino.
//electronicsmith.com
#include <Servo.h> //include servo librarry
Servo myServo;
const int flexPin = A0; //connect flex pin to ardiuino pin A0
void setup()
{
myServo.attach(7); // connect servo pin to arduino pin 7
}
void loop()
{
int flexValue; //variable to store sensor value
int servoPosition;
flexValue = analogRead(flexPin);
servoPosition = map(flexValue, 800, 900, 0, 180);
servoPosition = constrain(servoPosition, 0, 180);
myServo.write(servoPosition);
delay(20);
}